InvalidatePathCache()
Call this if the bounding shape’s points change at runtime
Author Archives: Hang
[Unity Coding] Debug Physics2D.BoxCast
Physics2D.BoxCast(origin, size, angle, direction, distance)
Vector2 t_tL = origin+ new Vector2(-size.x * 0.5f, size.y * 0.5f);
Vector2 t_tR = origin+ new Vector2(size.x * 0.5f, size.y * 0.5f);
Vector2 t_bL = origin+ new Vector2(-size.x * 0.5f, -size.y * 0.5f);
Vector2 t_bR = origin+ new Vector2(size.x * 0.5f, -size.y * 0.5f);
Color t_lineColor = Color.cyan;
Debug.DrawLine(t_tL, t_tR, t_lineColor);
Debug.DrawLine(t_bL, t_bR, t_lineColor);
Debug.DrawLine(t_tL, t_bL, t_lineColor);
Debug.DrawLine(t_tR, t_bR, t_lineColor);
[Unity C#] Notes for ESC
Components
using Entitas;
using UnityEngine;
public class PositionComponent : IComponent {
public Vector3 value;
}
public class HealthComponent : IComponent {
public float value;
}
public sealed class DestroyedComponent : IComponent {}
InitializeSystem
public sealed class CreatePlayerSystem : IInitializeSystem {
readonly Contexts _contexts;
public CreatePlayerSystem (Contexts g_contexts) {
_contexts = g_contexts;
}
public void Initialize () {
GameEntity t_entity = _contexts.game.CreateEntity ();
t_entity.AddHealth (100);
}
}
ExecuteSystem
public sealed class LogHealthSystem : IExecuteSystem {
readonly IGroup<GameEntity> _entities;
public LogHealthSystem (Contexts contexts) {
_entities = contexts.game.GetGroup (GameMatcher.Health);
}
public void Execute () {
foreach (GameEntity f_entity in _entities) {
float f_health = f_entity.health.value;
UnityEngine.Debug.Log ("health: " + f_health);
}
}
}
ReactiveSystem
public sealed class HealthSystem : ReactiveSystem<GameEntity> {
public HealthSystem (Contexts contexts) : base (contexts.game) {
}
// trigger for certain component
protected override ICollector<GameEntity> GetTrigger (IContext<GameEntity> context) {
return context.CreateCollector (GameMatcher.Health);
}
// filter for things you want to check
protected override bool Filter (GameEntity entity) {
return entity.hasHealth;
}
protected override void Execute (List<GameEntity> entities) {
foreach (GameEntity f_entity in entities) {
if (f_entity.health.value <= 0)
f_entity.isDestroyed = true;
}
}
}
A Root for All Systems
public class RootSystems : Feature {
public RootSystems (Contexts g_contexts) {
Add (new CreatePlayerSystem (g_contexts));
Add (new LogHealthSystem (g_contexts));
Add (new HealthSystem (g_contexts));
Add (new DestroyEntitySystem (g_contexts));
}
}
To Call Systems
RootSystems _systems;
void Start () {
//Contexts t_contexts = new Contexts ();
//Contexts otherWayToGetContexts = Contexts.sharedInstance;
_systems = new RootSystems (Contexts.sharedInstance);
_systems.Initialize ();
}
private void Update () {
_systems.Execute ();
}
[Unity] XBOX ONE wireless controller mapping on Mac (10.14.1)
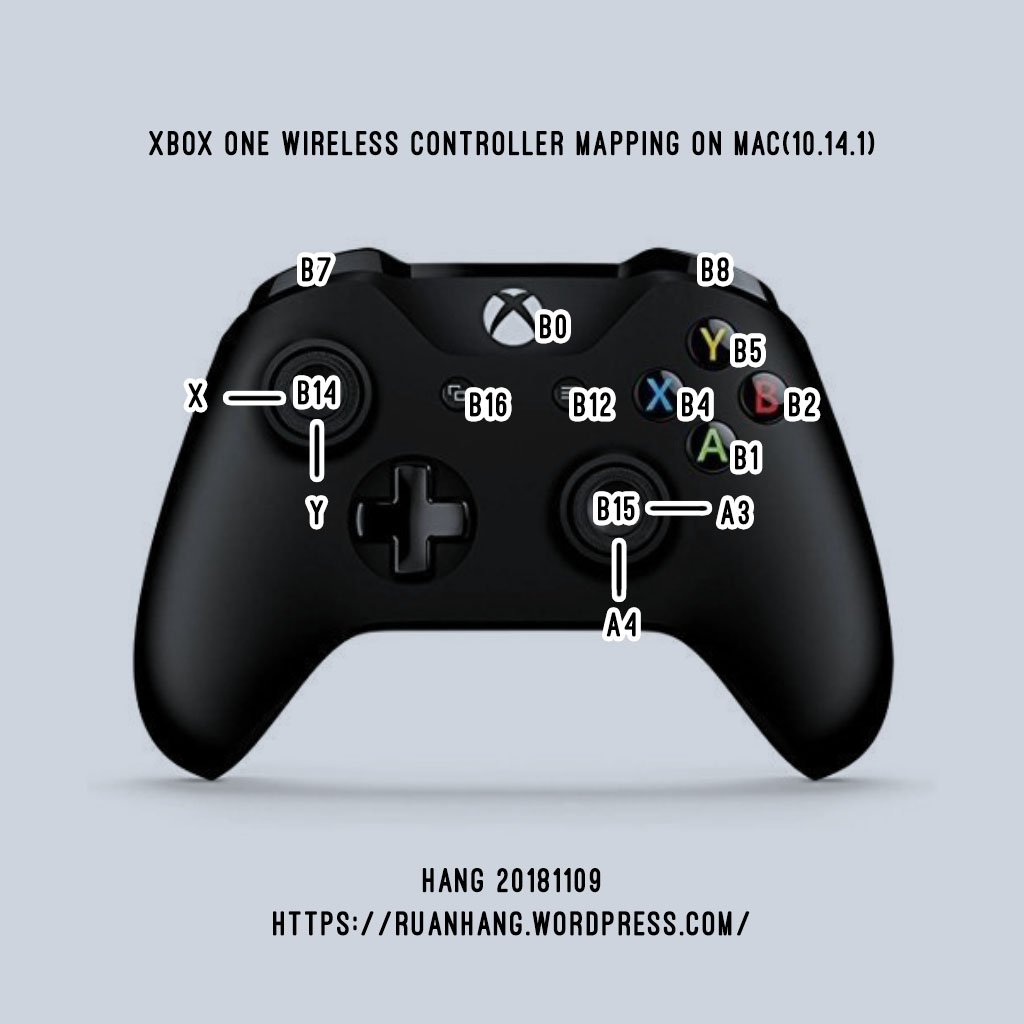
XBOX ONE wireless controller mapping on Mac (10.14.1) / Hang 20181109
[Unity Reminder] Quaternion
Quaternion.operator *
1) public static Quaternion operator *(Quaternion lhs, Quaternion rhs);
– Combines rotations lhs and rhs.
2) public static Vector3 operator *(Quaternion g_rotation, Vector3 g_point);
– Rotates the point g_point with g_rotation.
[Unity Reminder] Triplanar Shader
[Unity Reminder] Timeline!
[Unity Reminder] code I want to try
Boids
[Unity Reminder] Visual
Post Processing Stack